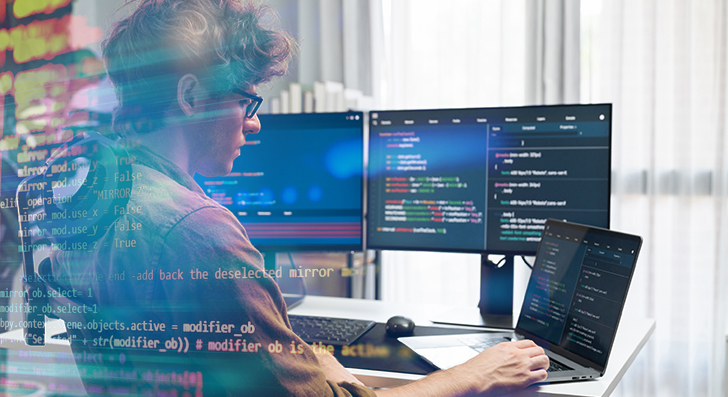
Scalability signifies your software can cope with progress—a lot more users, extra facts, plus much more traffic—with out breaking. To be a developer, making with scalability in mind will save time and pressure later. In this article’s a transparent and sensible guideline to assist you to get started by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is not some thing you bolt on afterwards—it should be component of your prepare from the beginning. Lots of programs fail if they expand fast mainly because the original style and design can’t deal with the additional load. As being a developer, you'll want to Believe early regarding how your process will behave under pressure.
Get started by planning your architecture to be versatile. Stay clear of monolithic codebases the place every thing is tightly linked. Instead, use modular layout or microservices. These styles break your application into lesser, independent areas. Each module or support can scale By itself without the need of affecting The entire technique.
Also, think about your database from day just one. Will it need to deal with 1,000,000 end users or merely 100? Choose the correct variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t will need them nonetheless.
An additional crucial place is to stay away from hardcoding assumptions. Don’t write code that only functions below existing problems. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven techniques. These support your app manage a lot more requests without having acquiring overloaded.
Once you Construct with scalability in mind, you are not just preparing for fulfillment—you happen to be cutting down foreseeable future complications. A effectively-planned method is easier to take care of, adapt, and improve. It’s greater to get ready early than to rebuild later.
Use the Right Databases
Picking out the appropriate database is really a key part of setting up scalable apps. Not all databases are constructed the same, and utilizing the Mistaken one can gradual you down as well as trigger failures as your application grows.
Start out by knowing your information. Can it be really structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. In addition they help scaling methods like browse replicas, indexing, and partitioning to deal with more targeted visitors and knowledge.
If your knowledge is more versatile—like person action logs, product catalogs, or paperwork—consider a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured facts and may scale horizontally more conveniently.
Also, take into account your study and write styles. Are you currently doing a great deal of reads with much less writes? Use caching and read replicas. Will you be handling a weighty write load? Explore databases that can manage significant create throughput, as well as party-primarily based knowledge storage devices like Apache Kafka (for temporary info streams).
It’s also clever to think forward. You may not need State-of-the-art scaling features now, but picking a databases that supports them usually means you received’t require to change later on.
Use indexing to hurry up queries. Prevent unnecessary joins. Normalize or denormalize your information according to your entry designs. And normally keep track of database efficiency while you increase.
In a nutshell, the appropriate databases will depend on your application’s framework, pace desires, And just how you hope it to mature. Take time to pick sensibly—it’ll save lots of difficulty later.
Optimize Code and Queries
Quick code is key to scalability. As your app grows, each little delay provides up. Inadequately published code or unoptimized queries can decelerate general performance and overload your procedure. That’s why it’s imperative that you Make successful logic from the start.
Begin by writing clear, uncomplicated code. Steer clear of repeating logic and take away anything avoidable. Don’t select the most intricate Remedy if a simple a person functions. Keep your functions short, focused, and straightforward to check. Use profiling equipment to seek out bottlenecks—areas exactly where your code requires much too prolonged to run or takes advantage of an excessive amount memory.
Up coming, take a look at your database queries. These frequently slow matters down much more than the code by itself. Be sure Every question only asks for the information you actually have to have. Keep away from Pick *, which fetches anything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Primarily across substantial tables.
Should you see the identical details becoming asked for again and again, use caching. Keep the results briefly applying resources like Redis or Memcached therefore you don’t have to repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application extra effective.
Remember to exam with large datasets. Code and queries that perform wonderful with a hundred documents may crash whenever they have to take care of one million.
In short, scalable apps are quickly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These steps assist your application remain sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more users and even more site visitors. If almost everything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources aid keep your app fast, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of just one server undertaking every one of the perform, the load balancer routes customers to various servers according to availability. This means no one server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Equipment like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge briefly so it can be reused immediately. When people request exactly the same information and facts yet again—like an item webpage or possibly a profile—you don’t ought to fetch it through the database every time. You can provide it from your cache.
There are two prevalent forms of caching:
1. Server-facet caching (like Redis or Memcached) merchants information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching decreases databases load, enhances velocity, and helps make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally ensure your cache is current when information does transform.
In brief, load balancing and caching are uncomplicated but potent equipment. Together, they help your application deal with far more users, remain rapid, and Get better from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app increase quickly. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you require them. You don’t really have to invest in hardware or guess long term capability. When targeted traffic boosts, you may insert extra means with just some clicks or quickly using vehicle-scaling. When traffic drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You could focus on building your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This can make it effortless to move your app concerning environments, from the laptop computer to the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
In short, working with cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when issues transpire. If you would like your application to grow with no restrictions, get started making use of these applications early. They conserve time, lower risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a key A part of constructing scalable devices.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently glitches transpire, and where by they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems fast, often before users even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you could roll it back again prior to it causes serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it really works well, even stressed.
Final Ideas
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Create applications Gustavo Woltmann news that expand efficiently with out breaking under pressure. Get started little, Consider significant, and Construct clever.